DefaultIfEmpty
emit items from the source Observable, or a default item if the source Observable emits nothing
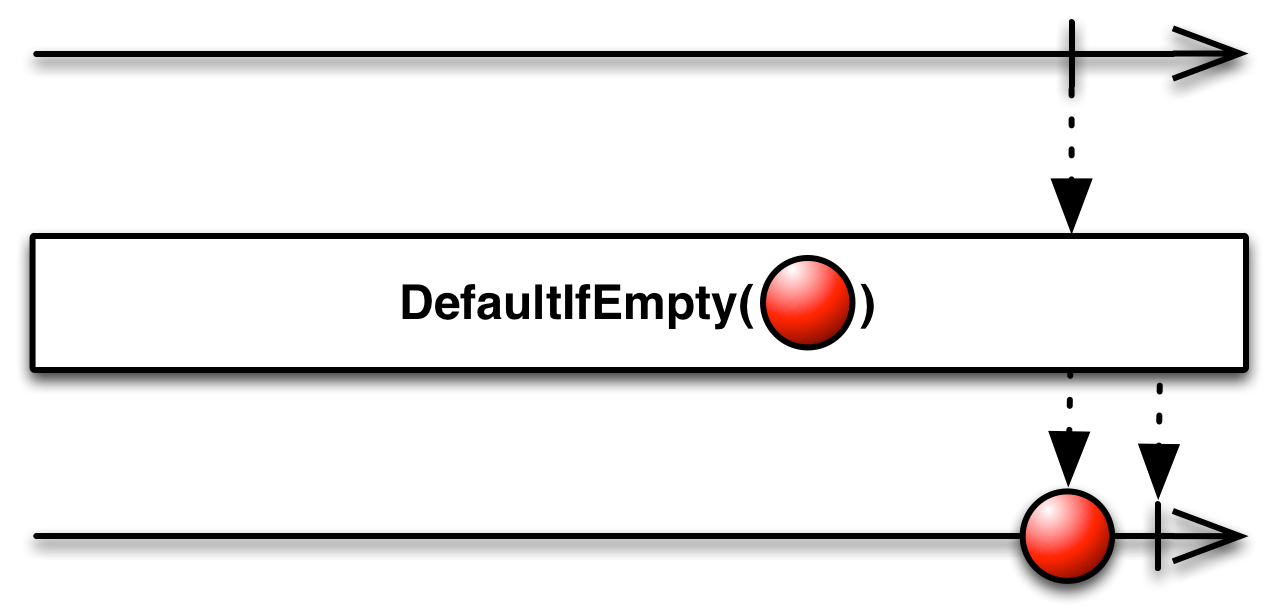
The DefaultIfEmpty operator simply mirrors the source Observable exactly if the source Observable emits any items. If the source Observable terminates normally (with an onComplete
) without emitting any items, the Observable returned from DefaultIfEmpty will instead emit a default item of your choosing before it too completes.
See Also
Language-Specific Information
RxGroovy defaultIfEmpty switchIfEmpty
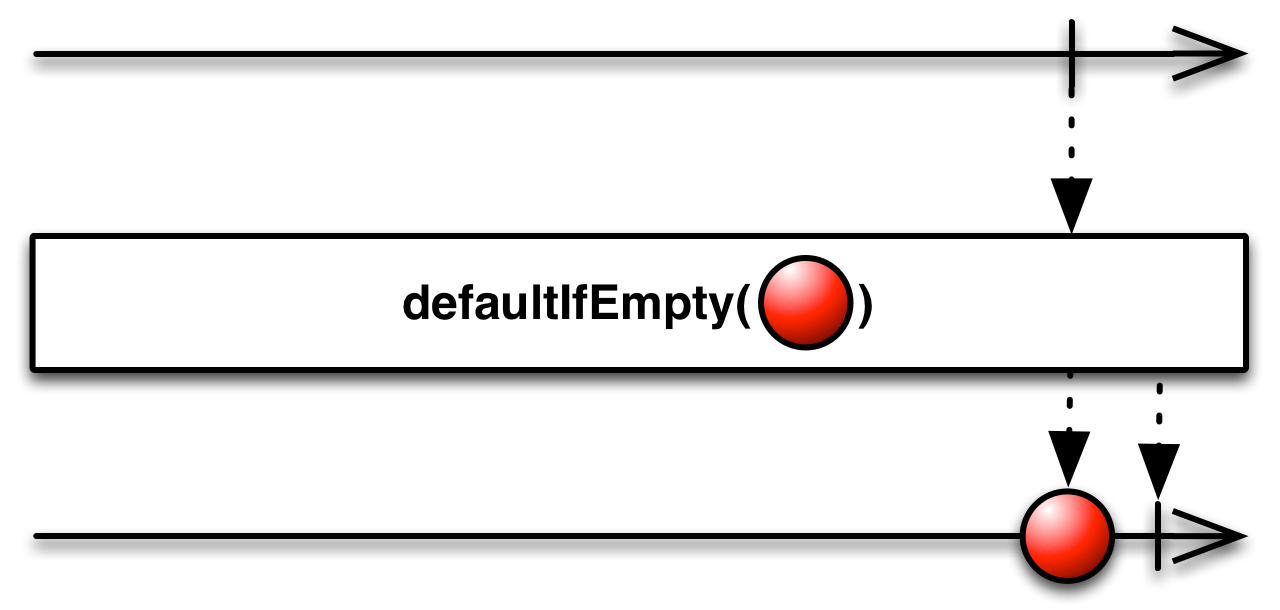
RxGroovy implements this operator as defaultIfEmpty
.
This operator does not by default operate on any particular Scheduler.
- Javadoc:
defaultIfEmpty(T)
There is also a new operator in RxGroovy 1.1 called switchIfEmpty
that, rather than emitting a backup value if the source Observable terminates without having emitted any items, it emits the emissions from a backup Observable.
RxJava 1․x defaultIfEmpty switchIfEmpty
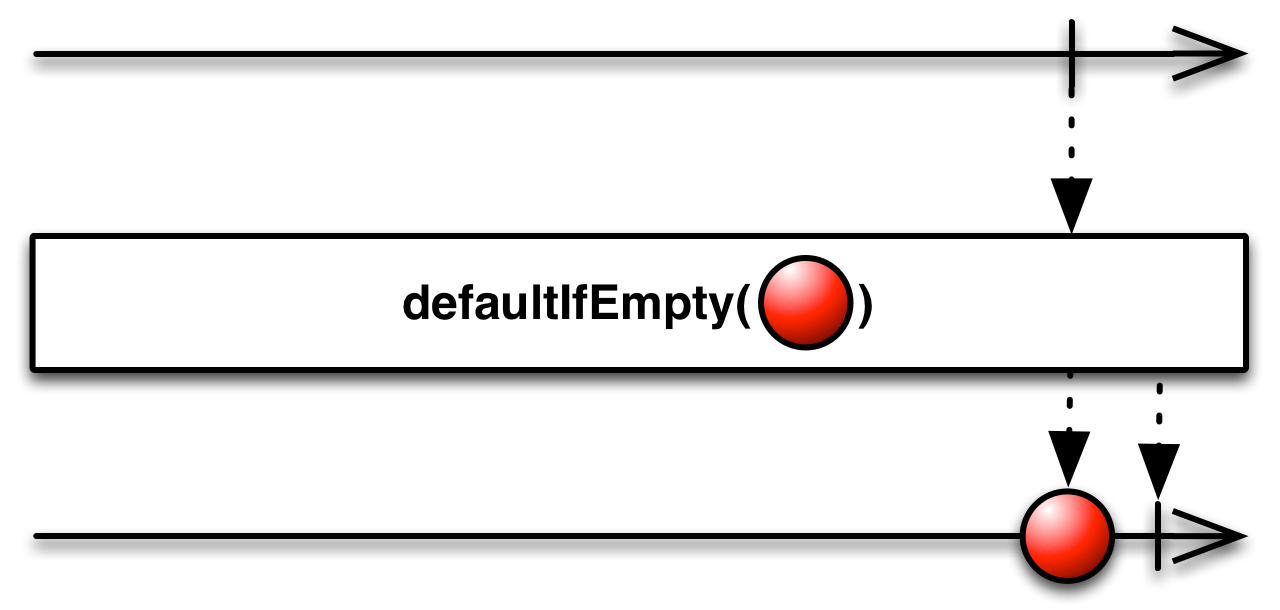
RxJava implements this operator as defaultIfEmpty
.
This operator does not by default operate on any particular Scheduler.
- Javadoc:
defaultIfEmpty(T)
There is also a new operator in RxJava 1.1 called switchIfEmpty
that, rather than emitting a backup value if the source Observable terminates without having emitted any items, it emits the emissions from a backup Observable.
Sample Code
Observable.empty().defaultIfEmpty(10).subscribe( val -> System.out.println("next: " + val), err -> System.err.println(err) , () -> System.out.println("completed") );
next: 10 completed
RxJava 2․x defaultIfEmpty switchIfEmpty
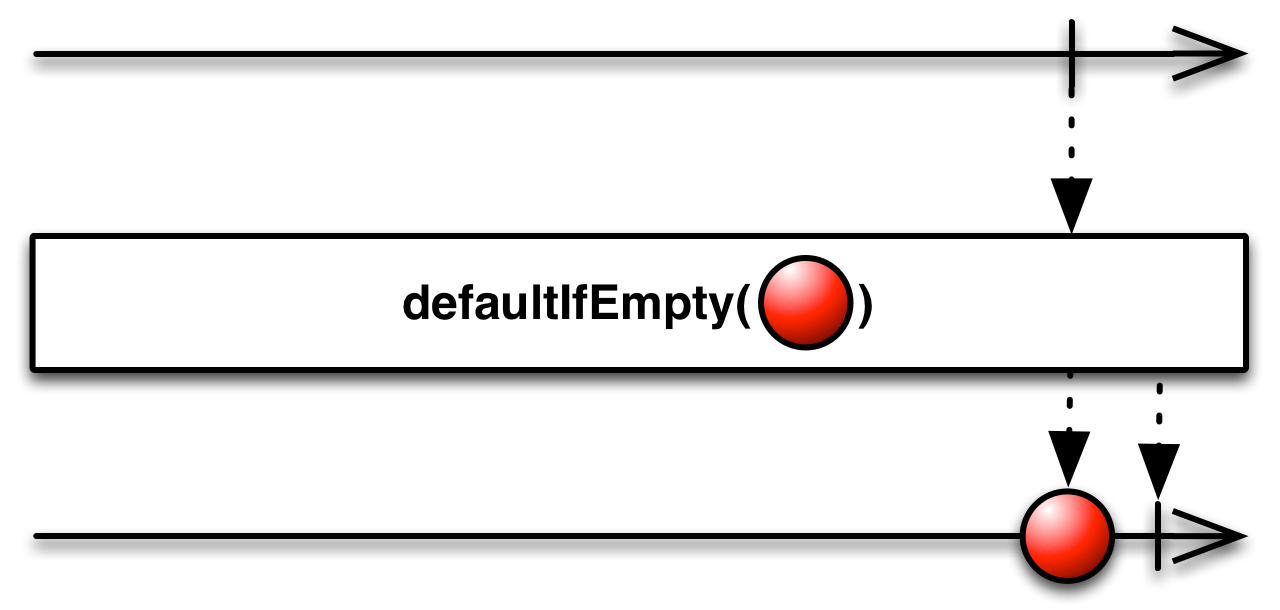
RxJava implements this operator as defaultIfEmpty
.
This operator does not by default operate on any particular Scheduler.
- Javadoc:
defaultIfEmpty(T)
Sample Code
Flowable.empty().defaultIfEmpty(10).subscribe( val -> System.out.println("next: " + val), err -> System.err.println(err) , () -> System.out.println("completed") );
next: 10 completed
RxJS defaultIfEmpty
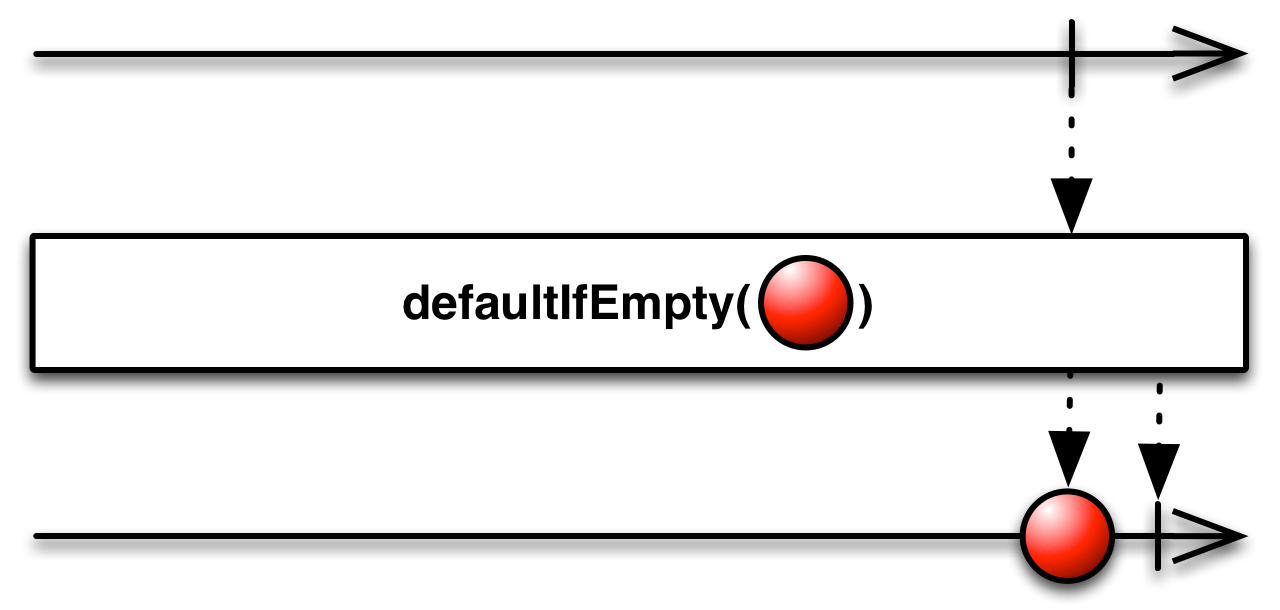
RxJS implements defaultIfEmpty
, but the parameter that sets the default value is optional. If you do not pass this default value, defaultIfEmpty
will emit a “null
” if the source Observable completes without emitting anything. (Note that an emission of a “null
” is not the same as no emission.)
Sample Code
/* Without a default value */ var source = Rx.Observable.empty().defaultIfEmpty(); var subscription = source.subscribe( function (x) { console.log('Next: ' + x.toString()); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); });
Next: null Completed
/* With a defaultValue */ var source = Rx.Observable.empty().defaultIfEmpty(false); var subscription = source.subscribe( function (x) { console.log('Next: ' + x.toString()); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); });
Next: false Completed
defaultIfEmpty
is found in each of the following distributions:
rx.js
rx.all.js
rx.all.compat.js
rx.compat.js
rx.lite.js
rx.lite.compat.js
RxPHP defaultIfEmpty
RxPHP implements this operator as defaultIfEmpty
.
Returns the specified value of an observable if the sequence is empty.
Sample Code
//from https://github.com/ReactiveX/RxPHP/blob/master/demo/defaultIfEmpty/defaultIfEmpty.php $source = \Rx\Observable::empty()->defaultIfEmpty(Rx\Observable::of('something')); $subscription = $source->subscribe($stdoutObserver);
Next value: something Complete!
© ReactiveX contributors
Licensed under the Apache License 2.0.
http://reactivex.io/documentation/operators/defaultifempty.html